When working with optional values and error handling in C#, developers often face choices between different approaches. This article focuses on comparing Nullable Reference Types, Option, and Result patterns to determine which fits best in various scenarios. From performance implications to explicit flow control and ease of use, we’ll break down each method to help you make informed decisions for your codebase.
Let’s evaluate these three patterns comprehensively across performance, complexity to use, potential developer mistakes, and explicit flow control and declarative styles.
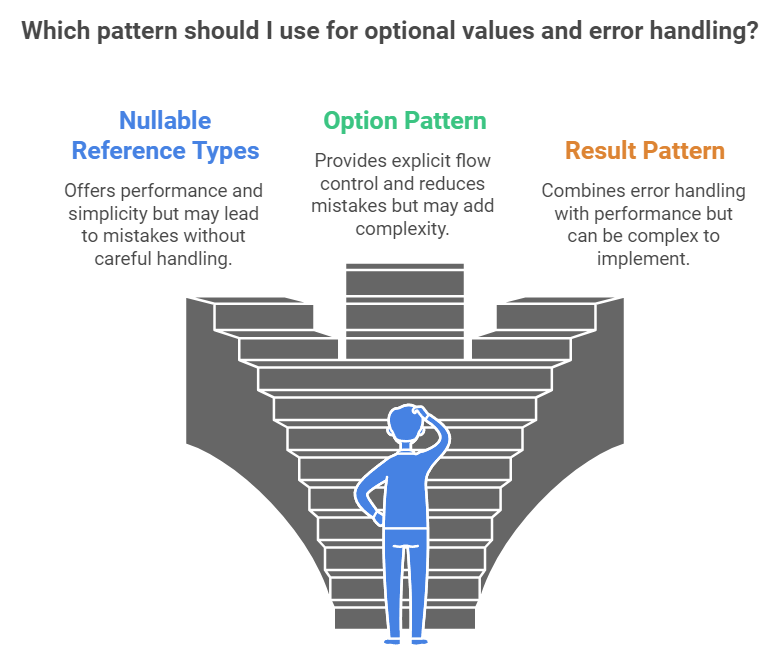
Quick Summary of Nullable Reference Types, Option, and Result
Nullable Reference Types (NRT): Introduced in C# 8.0, NRTs enhance null-safety by using compiler annotations to indicate whether a reference type can be null. They don’t add runtime overhead and are useful for maintaining backward compatibility with existing null-handling practices.
Option<T>: A functional programming construct representing a value that is either present (Some
) or absent (None
). It explicitly avoids null
, making optionality more robust and expressive. While not natively supported in C#, it’s commonly used through third-party libraries.
Result<T, E>: Like Option<T>, the Result pattern is functional programming construct and designed to encapsulate the success or failure of an operation. It combines a result value (T
) and an error state (E
), enforcing explicit handling of both scenarios. This pattern is widely used to avoid exceptions and provide structured error handling.
1. Nullable Reference Types (NRT)
Performance
- Memory: No additional memory overhead. NRTs are purely compile-time constructs.
- Runtime Cost: Minimal. Null-checks already exist in .NET’s runtime and are highly optimized.
- Garbage Collection (GC): Standard GC behavior applies to nullable objects. No additional allocations.
Complexity to Use
- Ease of Adoption: Very easy for existing .NET developers since nullability is intrinsic to the language.
- Tooling Support: Full IDE/compiler support (e.g., warnings for unhandled nullable references).
- Code Simplicity: Minimal boilerplate; no explicit wrapping/unwrapping needed.
Potential Mistakes
- Developer Misunderstanding:
- Ignoring or suppressing nullable warnings may lead to null-reference exceptions.
- Developers might forget to handle null explicitly if they rely solely on annotations.
- Implicit Assumptions:
- It’s possible to bypass the nullable annotations entirely by using unannotated legacy APIs.
Explicit Flow Control & Declarative Style
- Explicitness: NRTs rely on compiler-enforced contracts, but the flow control is not explicit.
- Example:
string? GetName() => null;
if (name != null) Console.WriteLine(name.Length); // Implicit null-check
- Declarative Style: Weak declarative semantics. Null handling requires imperative checks or patterns.
2. Option<T>
Performance
- Memory:
- Typically a value type (struct) with a boolean and an optional value field. This introduces minor overhead for wrapping but avoids allocations.
- Additional memory cost if used with reference types compared to NRT.
- Runtime Cost:
- Slightly higher than NRT because every operation (e.g.,
IsSome
) involves an additional check. - Accessing a value might require unwrapping (e.g.,
option.Match(...)
or similar).
- Slightly higher than NRT because every operation (e.g.,
- Garbage Collection (GC):
- If wrapping reference types,
Option<T>
still requires GC for the underlying type.
- If wrapping reference types,
Complexity to Use
- Ease of Adoption:
- Requires understanding functional paradigms.
- Not native in C#, so usage often depends on third-party libraries like
LanguageExt
.
- Tooling Support:
- No built-in tooling in .NET; developers must rely on library-specific APIs.
- Code Simplicity:
- Explicit wrapping/unwrapping (e.g.,
Some
/None
or pattern matching) adds complexity. - Example of checking and unwrapping:
- Explicit wrapping/unwrapping (e.g.,
Option<string> name = Option<string>.Some("Alice");
name.Match(
some => Console.WriteLine(some.Length),
none => Console.WriteLine("No name provided")
);
Potential Mistakes
- Developer Misunderstanding:
- Forgetting to handle both
Some
andNone
cases leads to incomplete logic. - Misusing it as a nullable substitute without leveraging the benefits of functional flow.
- Forgetting to handle both
- Implicit Assumptions:
- Developers might abuse
.Value
or similar APIs to retrieve the value directly, leading to runtime exceptions.
- Developers might abuse
Explicit Flow Control & Declarative Style
- Explicitness:
Option<T>
enforces explicit handling of absence through APIs likeMatch
or pattern matching. - Declarative Style: Stronger declarative semantics. Clear handling of value presence or absence.
- Example:
name.Match(
some => Console.WriteLine($"Name: {some}"),
none => Console.WriteLine("Name not provided.")
);
3. Result<T, E>
Performance
- Memory:
- Slightly heavier than
Option<T>
because it stores both a value (T) and an error (E). - Struct-based implementations avoid allocations but increase stack pressure.
- Slightly heavier than
- Runtime Cost:
- Accessing values involves checks for both success (
IsSuccess
) and error states. - Pattern matching or explicit unwrapping can add small overhead.
- Accessing values involves checks for both success (
- Garbage Collection (GC):
- If used with reference types, GC applies to the underlying types.
Complexity to Use
- Ease of Adoption:
- Requires understanding of functional or domain-driven design concepts.
- Explicit wrapping/unwrapping and success/error handling can feel verbose.
- Tooling Support:
- No built-in support in C#; typically requires third-party libraries.
- Code Simplicity:
- Often more verbose due to explicit success/error handling.
- Example of handling both states:
Result<string, string> result = GetUserName(userId);
result.Match(
success => Console.WriteLine($"User: {success}"),
error => Console.WriteLine($"Error: {error}")
);
Potential Mistakes
- Developer Misunderstanding:
- Misusing
Result
as a nullable substitute, ignoring its purpose for error handling. - Improper or incomplete handling of success and error cases leads to logic gaps.
- Misusing
- Implicit Assumptions:
- Misuse of
.Value
without checking for error state can result in runtime exceptions.
- Misuse of
Explicit Flow Control & Declarative Style
- Explicitness: The pattern enforces handling success/failure states explicitly.
- Declarative Style: Strong declarative semantics. The flow of control is clear and prevents hidden null/reference states.
- Example:
result.Match(
success => Console.WriteLine($"User: {success}"),
error => Console.WriteLine($"Error: {error}")
);
Summary Table
Aspect | NRT | Option<T> | Result<T, E> |
---|---|---|---|
Performance | Zero runtime overhead | Slight wrapping overhead | Slightly heavier than Option<T> |
Memory Overhead | None | Minimal (struct-based) | Moderate (tracks value + error) |
Complexity to Use | Low | Moderate | High |
Potential Mistakes | Ignored nullability warnings | Misusing .Value directly | Ignoring error-handling paths |
Explicitness | Weak (implicit null checks) | Strong | Very Strong |
Declarative Style | Minimal | Clear presence/absence handling | Explicit success/error handling |
When to Use Each
- NRT:
- Use for general absence/presence tracking where runtime performance and simplicity are critical.
- Ideal for legacy APIs or where null is a natural part of the domain.
- Option<T>:
- Use when null is unacceptable, and you need explicit handling of presence/absence but without error details.
- Best for functional programming scenarios or clear modeling of optionality.
- Result<T, E>:
- Use for domains requiring clear success/failure states with detailed error handling.
- Best for APIs where you want to avoid exceptions and ensure structured error recovery.
Wrapping up
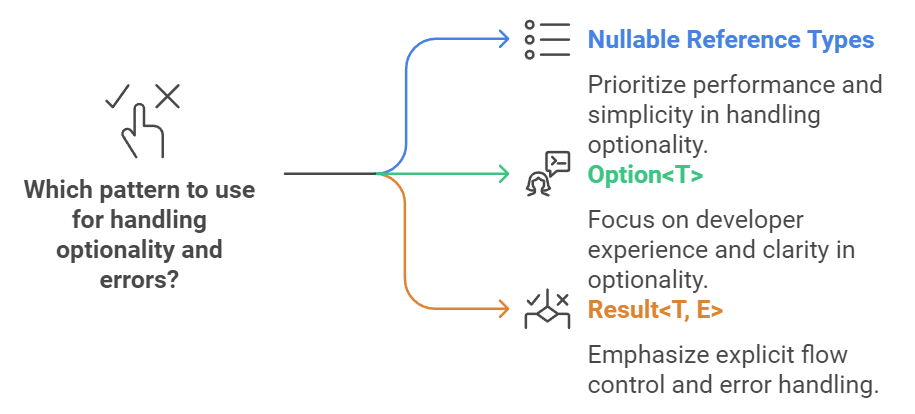
In conclusion, comparing Nullable Reference Types, Option, and Result reveals distinct strengths and trade-offs for handling optionality and errors in C#. Whether you prioritize performance, developer experience, or explicit flow control, each pattern has its place. Which approach do you prefer in your projects? Join the discussion below and share your thoughts or experiences with these patterns!